Dibi - smart database layer for PHP 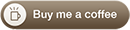
Database access functions in PHP are not standardised. This library hides the differences between them, and above all, it gives you a very handy interface.
The best way to install Dibi is to use a Composer:
php composer.phar require dibi/dibi
Or you can download the latest package from https://dibiphp.com. In this
package is also Dibi.minified
, shrinked single-file version of whole Dibi,
useful when you don't want to modify the library, but just use it.
Dibi requires PHP 5.4.4 or later. It has been tested with PHP 7 too.
Refer to the examples
directory for examples. Dibi documentation is
available on the homepage.
Connect to database:
// connect to database (static way)
dibi::connect([
'driver' => 'mysql',
'host' => 'localhost',
'username' => 'root',
'password' => '***',
]);
// or object way; in all other examples use $connection-> instead of dibi::
$connection = new DibiConnection($options);
SELECT, INSERT, UPDATE
dibi::query('SELECT * FROM users WHERE id = ?', $id);
$arr = [
'name' => 'John',
'is_admin' => TRUE,
];
dibi::query('INSERT INTO users', $arr);
// INSERT INTO users (`name`, `is_admin`) VALUES ('John', 1)
dibi::query('UPDATE users SET', $arr, 'WHERE `id`=?', $x);
// UPDATE users SET `name`='John', `is_admin`=1 WHERE `id` = 123
dibi::query('UPDATE users SET', [
'title' => array('SHA1(?)', 'tajneheslo'),
]);
// UPDATE users SET 'title' = SHA1('tajneheslo')
Getting results
$result = dibi::query('SELECT * FROM users');
$value = $result->fetchSingle(); // single value
$all = $result->fetchAll(); // all rows
$assoc = $result->fetchAssoc('id'); // all rows as associative array
$pairs = $result->fetchPairs('customerID', 'name'); // all rows as key => value pairs
// iterating
foreach ($result as $n => $row) {
print_r($row);
}
Modifiers for arrays:
dibi::query('SELECT * FROM users WHERE %and', [
array('number > ?', 10),
array('number < ?', 100),
]);
// SELECT * FROM users WHERE (number > 10) AND (number < 100)
%and | `[key]=val AND [key2]="val2" AND ...` | |
%or | `[key]=val OR [key2]="val2" OR ...` | |
%a | assoc | `[key]=val, [key2]="val2", ...` |
%l %in | list | `(val, "val2", ...)` |
%v | values | `([key], [key2], ...) VALUES (val, "val2", ...)` |
%m | multivalues | `([key], [key2], ...) VALUES (val, "val2", ...), (val, "val2", ...), ...` |
%by | ordering | `[key] ASC, [key2] DESC ...` |
%n | identifiers | `[key], [key2] AS alias, ...` |
other | - | `val, val2, ...` |
Modifiers for LIKE
dibi::query("SELECT * FROM table WHERE name LIKE %like~", $query);
%like~ | begins with |
%~like | ends with |
%~like~ | contains |
DateTime:
dibi::query('UPDATE users SET', [
'time' => new DateTime,
]);
// UPDATE users SET ('2008-01-01 01:08:10')
Testing:
echo dibi::$sql; // last SQL query
echo dibi::$elapsedTime;
echo dibi::$numOfQueries;
echo dibi::$totalTime;