Thanks for this great plugin. Here is an issue I've encountered:
Platform
[x] iOS
[?: Not tested] Android
Expected behavior
onAdClosed
should fire each time an interstitial ad is closed.
Actual behavior
I am experiencing a situation where onAdClosed
is not firing when closing an interstitial ad. The miss-fire occurs seemingly randomly, and the exact occurrence within a repeated series of preloading then showing interstitial ads is not able to be reproduced, but if repeated long enough it will eventually occur reliably.
The ad is preloaded as instructed in the README, and shown after it has correctly loaded, also as documented.
The bug has been reproduced:
- both an iOS simulator (iPhone 6) and a physical device (iPhone 6S+), both running iOS 11
- both in the demo app and another app that I'm building
Additional notes
The delightful output shown a couple sections below is from the demo app, undergoing the sequence described here, following app launch.
First Cycle:
- "preload interstitial" button clicked. Wait for the "Interstitial Preloaded" message to show.
- "show interstitial" button clicked.
- Watch the ad, then click the "x" symbol to close it.
- Observe the "onAdClosed called" message (I changed the text of that message to be easier to search in the logs)
Second Cycle:
- "preload interstitial" button clicked. Wait for the "Interstitial Preloaded" message to show.
- "show interstitial" button clicked.
- Watch the ad, then click the "x" symbol to close it.
- "onAdClosed called" message never displays, instead the (usually hidden) "Interstitial Showing" message remains showing.
Note that the next interstitial can still then be preloaded as expected, and the interstitial can again be shown. onAdClosed
then may, or may not fire - I have observed both.
Screenshots showing the failed state for both emulator and physical device
Note the "Interstitial Showing" message that is partially hidden. That always happens when this bug happens - perhaps it's a clue. All other app messages are not obscured.
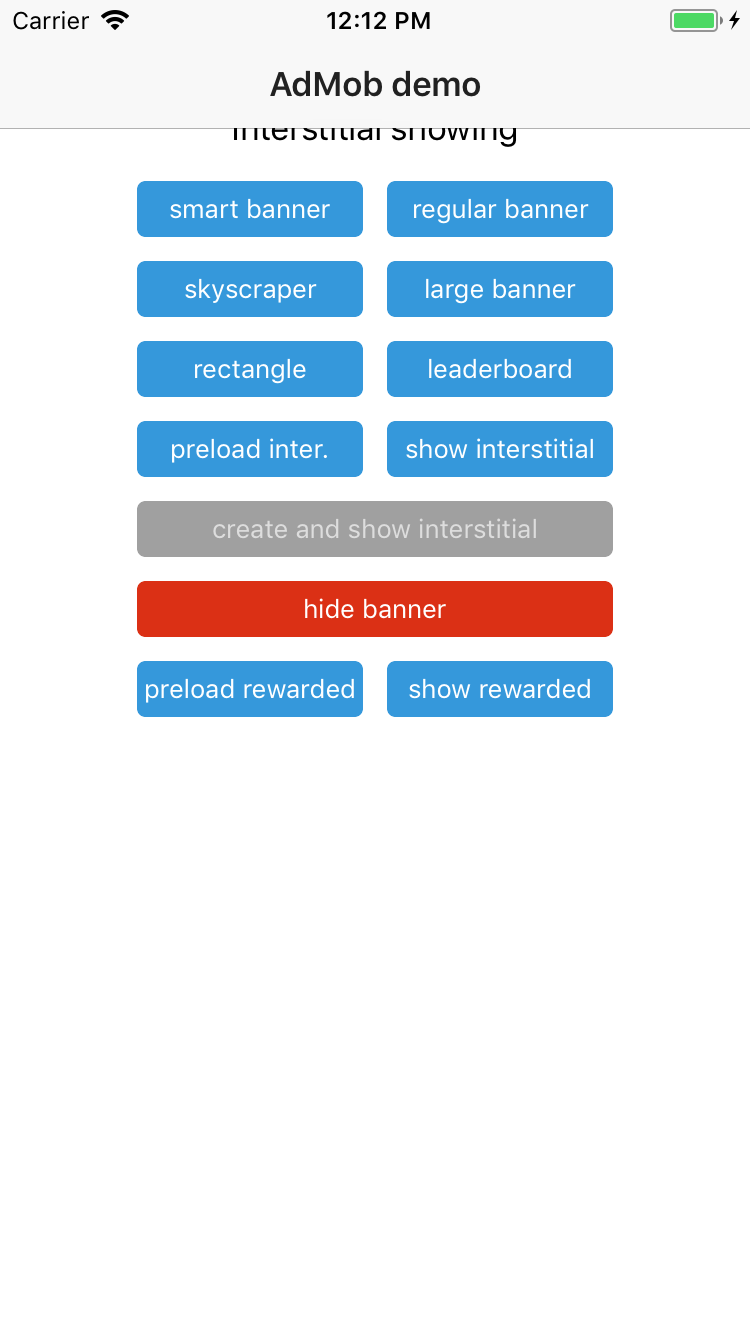
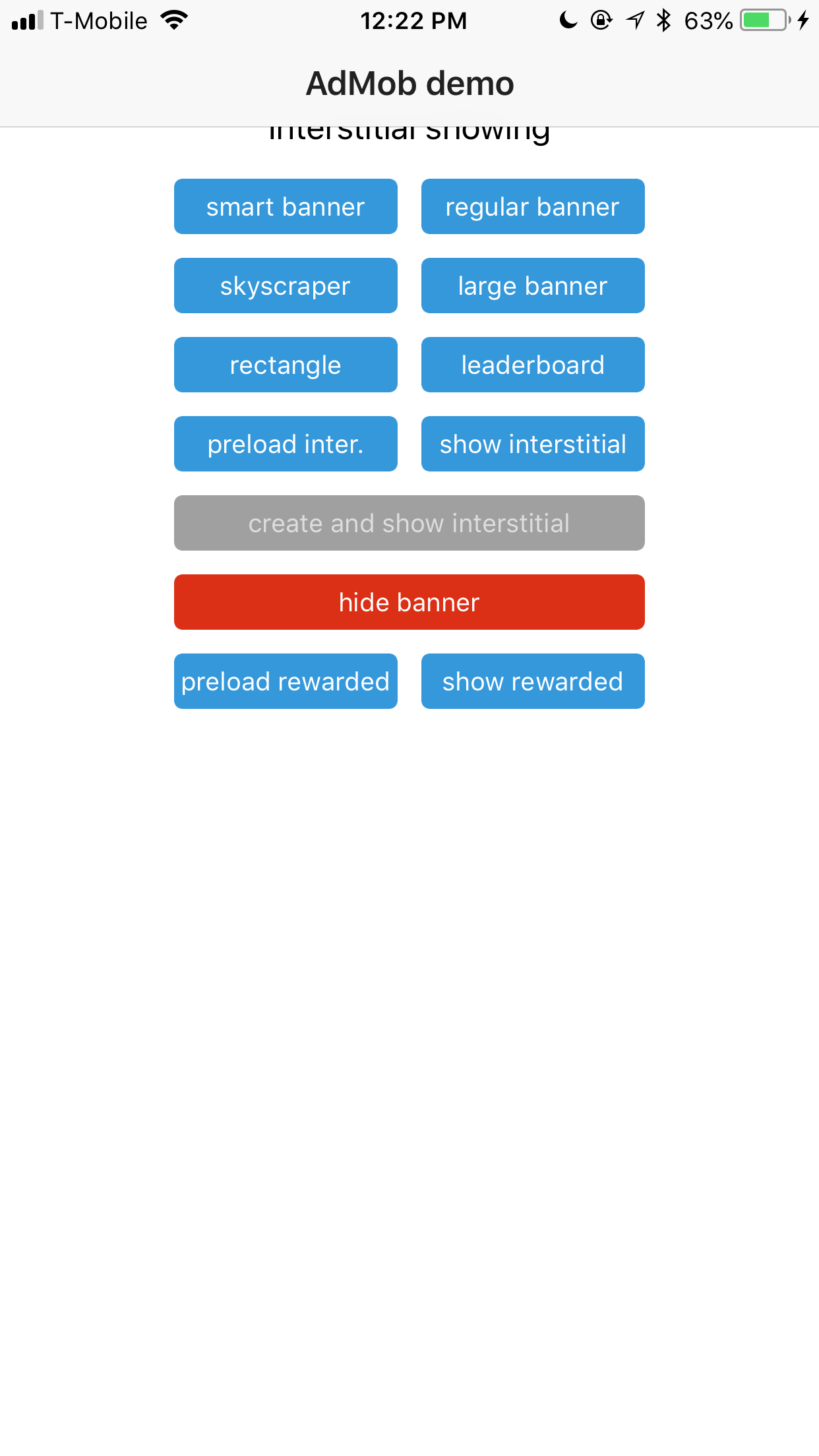
Logs from physical device
Successfully synced application org.nativescript.admobdemo on device XXXXXXXX-XXXX-XXX-XXXXX-XXXXXXXXXXXX.
0x10285a618 - UIProcess is taking a foreground assertion because the view is visible
0x1078e7000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x1072d0f00, main = 1)
Memory usage info dump at MainFrameLoadCompleted:
document_count: 1
virtual_size: 1745027072
internal: 34439168
javascript_gc_heap_capacity: 65536
resident_size: 75513856
phys_footprint: 34620016
pagecache_page_count: 0
compressed: 0
javascript_gc_heap_extra_memory_size: 0
Memory usage info dump at MainFrameLoadStarted:
document_count: 1
virtual_size: 1748942848
internal: 35094528
javascript_gc_heap_capacity: 65536
resident_size: 78888960
phys_footprint: 35308184
pagecache_page_count: 0
compressed: 0
javascript_gc_heap_extra_memory_size: 0
0x15de0f6a0 - FrameLoader::prepareForLoadStart: Starting frame load (frame = 0x105804300, main = 1)
0x105c88000 - DocumentLoader::startLoadingMainResource: Starting load (frame = 0x105804300, main = 1)
Faulting in NSHTTPCookieStorage singleton
Faulting in CFHTTPCookieStorage singleton
Creating default cookie storage with default identifier
TIC Enabling TLS [1:0x1c017cf80]
TIC TCP Conn Start [1:0x1c017cf80]
Task .<0> setting up Connection 1
[1 ] start
TIC TLS Event [1:0x1c017cf80]: 1, Pending(0)
TIC TLS Event [1:0x1c017cf80]: 2, Pending(0)
TIC TLS Event [1:0x1c017cf80]: 11, Pending(0)
TIC TLS Event [1:0x1c017cf80]: 12, Pending(0)
TIC TLS Event [1:0x1c017cf80]: 14, Pending(0)
TIC TLS Trust Result [1:0x1c017cf80]: 0
TIC TLS Event [1:0x1c017cf80]: 20, Pending(0)
TIC TCP Conn Connected [1:0x1c017cf80]: Err(16)
TIC TCP Conn Event [1:0x1c017cf80]: 1
TIC TCP Conn Event [1:0x1c017cf80]: 8
TIC TLS Handshake Complete [1:0x1c017cf80]
Task .<0> now using Connection 1
Task .<0> sent request, body N
Task .<0> received response, status 200 content C
Task .<0> response ended
Task <5BEB8852-CD58-4FC0-88D1-4D1F8D1C8D70>.<0> now using Connection 1
Task <5BEB8852-CD58-4FC0-88D1-4D1F8D1C8D70>.<0> sent request, body N
Task <5BEB8852-CD58-4FC0-88D1-4D1F8D1C8D70>.<0> received response, status 200 content K
Task <5BEB8852-CD58-4FC0-88D1-4D1F8D1C8D70>.<0> response ended
Task .<0> now using Connection 1
Task .<0> sent request, body N
TIC Enabling TLS [2:0x1c417de80]
TIC TCP Conn Start [2:0x1c417de80]
Task .<0> setting up Connection 2
[2 ] start
Task .<0> received response, status 200 content K
TIC TLS Event [2:0x1c417de80]: 1, Pending(0)
Task .<0> response ended
TIC TLS Event [2:0x1c417de80]: 2, Pending(0)
TIC TLS Event [2:0x1c417de80]: 20, Pending(0)
TIC TCP Conn Connected [2:0x1c417de80]: Err(16)
TIC TCP Conn Event [2:0x1c417de80]: 1
TIC TCP Conn Event [2:0x1c417de80]: 8
TIC TLS Handshake Complete [2:0x1c417de80]
Task .<0> now using Connection 2
Task .<0> sent request, body N
Task .<0> received response, status 200 content K
Task .<0> response ended
Memory usage info dump at MainFrameLoadCompleted:
document_count: 2
virtual_size: 1792196608
internal: 46563328
javascript_gc_heap_capacity: 3371088
resident_size: 92454912
phys_footprint: 46531264
pagecache_page_count: 0
compressed: 0
javascript_gc_heap_extra_memory_size: 0
0x15de0f6a0 - FrameLoader::checkLoadCompleteForThisFrame: Finished frame load (frame = 0x105804300, main = 1)
0x10789b000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x105cddb00, main = 0)
0x107193000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x1058a7840, main = 0)
Task .<0> now using Connection 1
Task .<0> sent request, body N
0x107191000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10879a400, main = 0)
Task .<0> received response, status 200 content C
Task .<0> response ended
0x107190000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x108798000, main = 0)
0x108984000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10879b3c0, main = 0)
0x108986000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10874c6c0, main = 0)
TIC Enabling TLS [3:0x1c417e540]
TIC TCP Conn Start [3:0x1c417e540]
Task .<1> setting up Connection 3
[3 ] start
0x108985000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10874d200, main = 0)
TIC TLS Event [3:0x1c417e540]: 1, Pending(0)
0x108987000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10874db00, main = 0)
TIC TLS Event [3:0x1c417e540]: 2, Pending(0)
TIC TLS Event [3:0x1c417e540]: 11, Pending(0)
TIC TLS Event [3:0x1c417e540]: 12, Pending(0)
TIC TLS Event [3:0x1c417e540]: 14, Pending(0)
TIC TLS Trust Result [3:0x1c417e540]: 0
TIC TLS Event [3:0x1c417e540]: 20, Pending(0)
TIC TCP Conn Connected [3:0x1c417e540]: Err(16)
TIC TCP Conn Event [3:0x1c417e540]: 1
TIC TCP Conn Event [3:0x1c417e540]: 8
TIC TLS Handshake Complete [3:0x1c417e540]
Task .<1> now using Connection 3
Task .<1> sent request, body N
Task .<1> received response, status 200 content K
Task .<1> response ended
0x108968000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x108bd6880, main = 0)
0x10785f000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x105807000, main = 1)
Memory usage info dump at MainFrameLoadCompleted:
document_count: 12
virtual_size: 1813495808
internal: 54738944
javascript_gc_heap_capacity: 5271836
resident_size: 101171200
phys_footprint: 54706920
pagecache_page_count: 0
compressed: 0
javascript_gc_heap_extra_memory_size: 1068
Memory usage info dump at MainFrameLoadStarted:
document_count: 12
virtual_size: 1813626880
internal: 55066624
javascript_gc_heap_capacity: 5271836
resident_size: 101892096
phys_footprint: 55034600
pagecache_page_count: 0
compressed: 0
javascript_gc_heap_extra_memory_size: 1068
0x15dda1f80 - FrameLoader::prepareForLoadStart: Starting frame load (frame = 0x105807000, main = 1)
0x108bb1000 - DocumentLoader::startLoadingMainResource: Returning cached main resource (frame = 0x105807000, main = 1)
Task <4B7F2CB4-BA10-47E8-8A5E-E409E6130367>.<0> now using Connection 1
Task <4B7F2CB4-BA10-47E8-8A5E-E409E6130367>.<0> sent request, body N
TIC Enabling TLS [4:0x1c0367800]
TIC TCP Conn Start [4:0x1c0367800]
Task <819F2372-0F4C-433D-A825-B7E2C0BFEA01>.<0> setting up Connection 4
[4 ] start
Task <4B7F2CB4-BA10-47E8-8A5E-E409E6130367>.<0> received response, status 200 content K
Task <4B7F2CB4-BA10-47E8-8A5E-E409E6130367>.<0> response ended
0x10aacd000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10aaeb300, main = 1)
TIC TLS Event [4:0x1c0367800]: 1, Pending(0)
0x10b8e8000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b8cfcc0, main = 0)
0x10b8ea000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10aae9800, main = 1)
0x10b8e9000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b8ce1c0, main = 0)
0x10b87d000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b8cd200, main = 0)
0x10b87f000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b8cdf80, main = 0)
0x10b87e000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b8cfa80, main = 0)
0x10b87c000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b86c6c0, main = 0)
0x10b861000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b86cfc0, main = 0)
0x10b863000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b86d8c0, main = 0)
TIC TLS Event [4:0x1c0367800]: 2, Pending(0)
0x10b862000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b86e1c0, main = 0)
0x10b860000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b86eac0, main = 0)
0x10b84d000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b86f3c0, main = 0)
0x10b84f000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b86fcc0, main = 0)
TIC TLS Event [4:0x1c0367800]: 11, Pending(0)
TIC TLS Event [4:0x1c0367800]: 12, Pending(0)
TIC TLS Event [4:0x1c0367800]: 14, Pending(0)
TIC TLS Trust Result [4:0x1c0367800]: 0
0x10b84e000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b86dd40, main = 0)
0x10b84c000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b86ef40, main = 0)
0x10b815000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b8206c0, main = 0)
TIC TLS Event [4:0x1c0367800]: 20, Pending(0)
TIC TCP Conn Connected [4:0x1c0367800]: Err(16)
TIC TCP Conn Event [4:0x1c0367800]: 1
TIC TCP Conn Event [4:0x1c0367800]: 8
TIC TLS Handshake Complete [4:0x1c0367800]
Task <819F2372-0F4C-433D-A825-B7E2C0BFEA01>.<0> now using Connection 4
Task <819F2372-0F4C-433D-A825-B7E2C0BFEA01>.<0> sent request, body N
0x10b817000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b820fc0, main = 0)
0x10b816000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b8218c0, main = 0)
0x10b814000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b8221c0, main = 0)
Task <819F2372-0F4C-433D-A825-B7E2C0BFEA01>.<0> received response, status 200 content K
Task <819F2372-0F4C-433D-A825-B7E2C0BFEA01>.<0> response ended
0x10bcf1000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b822d00, main = 0)
Memory usage info dump at MainFrameLoadCompleted:
document_count: 35
virtual_size: 1865187328
internal: 74596352
javascript_gc_heap_capacity: 11608096
resident_size: 123191296
phys_footprint: 74498832
pagecache_page_count: 0
compressed: 0
javascript_gc_heap_extra_memory_size: 33952
0x15dda1f80 - FrameLoader::checkLoadCompleteForThisFrame: Finished frame load (frame = 0x105807000, main = 1)
0x10bcf3000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b821440, main = 0)
0x10bcf2000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b823a80, main = 0)
0x10bcf0000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b86cb40, main = 0)
0x10bc91000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10bc94240, main = 0)
0x10bc93000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10bc94fc0, main = 0)
0x10bc92000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10bc97cc0, main = 0)
0x10bc90000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10bc961c0, main = 0)
0x10bc39000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10bc96ac0, main = 0)
0x10bc31000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10bc95f80, main = 0)
TIC Enabling TLS [5:0x1c417fec0]
TIC TCP Conn Start [5:0x1c417fec0]
Task <4FDBC7DC-9F83-47F7-A576-926E1BF9F2D2>.<1> setting up Connection 5
[5 ] start
0x10bc33000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10bc97a80, main = 0)
0x10bc3d000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10bc20240, main = 0)
0x10bc3e000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10bc20b40, main = 0)
0x10bc3f000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10bc21440, main = 0)
TIC TLS Event [5:0x1c417fec0]: 1, Pending(0)
TIC TLS Event [5:0x1c417fec0]: 2, Pending(0)
TIC TLS Event [5:0x1c417fec0]: 11, Pending(0)
TIC TLS Event [5:0x1c417fec0]: 12, Pending(0)
TIC TLS Event [5:0x1c417fec0]: 14, Pending(0)
TIC TLS Trust Result [5:0x1c417fec0]: 0
TIC TLS Event [5:0x1c417fec0]: 20, Pending(0)
TIC TCP Conn Connected [5:0x1c417fec0]: Err(16)
TIC TCP Conn Event [5:0x1c417fec0]: 1
TIC TCP Conn Event [5:0x1c417fec0]: 8
TIC TLS Handshake Complete [5:0x1c417fec0]
Task <4FDBC7DC-9F83-47F7-A576-926E1BF9F2D2>.<1> now using Connection 5
Task <4FDBC7DC-9F83-47F7-A576-926E1BF9F2D2>.<1> sent request, body N
Task <4FDBC7DC-9F83-47F7-A576-926E1BF9F2D2>.<1> received response, status 303 content K
Task <4FDBC7DC-9F83-47F7-A576-926E1BF9F2D2>.<1> response ended
TIC Enabling TLS [6:0x1c0369540]
TIC TCP Conn Start [6:0x1c0369540]
Task <4FDBC7DC-9F83-47F7-A576-926E1BF9F2D2>.<1> setting up Connection 6
[6 ] start
TIC TLS Event [6:0x1c0369540]: 1, Pending(0)
TIC TLS Event [6:0x1c0369540]: 2, Pending(0)
TIC TLS Event [6:0x1c0369540]: 11, Pending(0)
TIC TLS Event [6:0x1c0369540]: 12, Pending(0)
TIC TLS Event [6:0x1c0369540]: 14, Pending(0)
TIC TLS Trust Result [6:0x1c0369540]: 0
TIC TLS Event [6:0x1c0369540]: 20, Pending(0)
TIC TCP Conn Connected [6:0x1c0369540]: Err(16)
TIC TCP Conn Event [6:0x1c0369540]: 1
TIC TCP Conn Event [6:0x1c0369540]: 8
TIC TLS Handshake Complete [6:0x1c0369540]
Task <4FDBC7DC-9F83-47F7-A576-926E1BF9F2D2>.<1> now using Connection 6
Task <4FDBC7DC-9F83-47F7-A576-926E1BF9F2D2>.<1> sent request, body N
Task <4FDBC7DC-9F83-47F7-A576-926E1BF9F2D2>.<1> received response, status 206 content K
0x10c1f0000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x105cdef40, main = 0)
0x10c1f1000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10bc21f80, main = 0)
0x10c1d5000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10bc22880, main = 0)
0x10c1d7000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10bc23180, main = 0)
TIC Enabling TLS [7:0x1c4364080]
TIC TCP Conn Start [7:0x1c4364080]
Task <776FB2C0-F06D-4E84-B929-D7CED9CC58DE>.<0> setting up Connection 7
[7 ] start
TIC TCP Conn Cancel [6:0x1c0369540]
Task <4FDBC7DC-9F83-47F7-A576-926E1BF9F2D2>.<1> response ended
[6 stream, pid: 2677, url: https://r3---sn-qxo7rn7e.googlevideo.com/videoplayback?id=9a85d42a0861d0f5&itag=22&source=youtube&requiressl=yes&mm=31&mn=sn-qxo7r, tls] cancelled
[6.1 17A82409-6D92-459D-A023-2A198692E58D .54653<->]
Connected Path: satisfied (Path is satisfied), interface: en0, ipv4, dns
Duration: 0.289s, DNS @0.000s took 0.013s, TCP @0.015s took 0.015s, TLS took 0.049s
bytes in/out: 1314946/1098, packets in/out: 950/3, rtt: 0.028s, retransmitted packets: 0, out-of-order packets: 0
TIC TCP Conn Destroyed [6:0x1c0369540]
TIC TCP Conn Cancel [5:0x1c417fec0]
[5 stream, pid: 2677, url: https://www.youtube.com/get_video?video_id=moXUKghh0PU&ts=1552243197&t=DdhP5LZEnQC3ygFxIczO3fnxStk&gad=1&br=1&itag=22,18, tls] cancelled
[5.1 5CDFB159-97E5-4C9E-8B28-2F90252722F8 .54652<->]
Connected Path: satisfied (Path is satisfied), interface: en0, ipv4, dns
Duration: 0.486s, DNS @0.000s took 0.010s, TCP @0.012s took 0.015s, TLS took 0.050s
bytes in/out: 4764/873, packets in/out: 9/10, rtt: 0.018s, retransmitted packets: 1, out-of-order packets: 0
0x10c1f2000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x105cdfcc0, main = 0)
TIC TCP Conn Destroyed [5:0x1c417fec0]
TIC Read Status [5:0x0]: 1:57
TIC Read Status [5:0x0]: 1:57
0x10c1d4000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10bc21d40, main = 0)
0x10c1f3000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10bc22400, main = 0)
TIC TLS Event [7:0x1c4364080]: 1, Pending(0)
TIC TLS Event [7:0x1c4364080]: 2, Pending(0)
TIC TLS Event [7:0x1c4364080]: 11, Pending(0)
TIC TLS Event [7:0x1c4364080]: 12, Pending(0)
TIC TLS Event [7:0x1c4364080]: 14, Pending(0)
TIC TLS Trust Result [7:0x1c4364080]: 0
TIC TLS Event [7:0x1c4364080]: 20, Pending(0)
TIC TCP Conn Connected [7:0x1c4364080]: Err(16)
TIC TCP Conn Event [7:0x1c4364080]: 1
TIC TCP Conn Event [7:0x1c4364080]: 8
TIC TLS Handshake Complete [7:0x1c4364080]
Task <776FB2C0-F06D-4E84-B929-D7CED9CC58DE>.<0> now using Connection 7
Task <776FB2C0-F06D-4E84-B929-D7CED9CC58DE>.<0> sent request, body N
Task <776FB2C0-F06D-4E84-B929-D7CED9CC58DE>.<0> received response, status 204 content K
Task <776FB2C0-F06D-4E84-B929-D7CED9CC58DE>.<0> response ended
XPC connection invalidated (daemon unloaded or disabled)
client.trigger:#N CCFG for cid 0x35 has # of profiles: 2
client.trigger:#N Random sample for 0x350002 is skip
client.trigger:#N Random sample for 0x350002 is skip
client.trigger:#N Random sample for 0x350002 is skip
client.trigger:#N Random sample for 0x350002 is skip
client.trigger:#N Random sample for 0x350002 is skip
client.trigger:#N Random sample for 0x350002 is skip
client.trigger:#N Random sample for 0x350002 is skip
client.trigger:#N Random sample for 0x350002 is skip
Task <86D02672-633E-48FE-A26A-22AAD7400414>.<0> now using Connection 7
Task <86D02672-633E-48FE-A26A-22AAD7400414>.<0> sent request, body N
Task <86D02672-633E-48FE-A26A-22AAD7400414>.<0> received response, status 204 content K
Task <86D02672-633E-48FE-A26A-22AAD7400414>.<0> response ended
TIC Enabling TLS [8:0x1c036a080]
TIC TCP Conn Start [8:0x1c036a080]
Task <640DC1A5-F732-4783-88F7-41FBCD5300B0>.<1> setting up Connection 8
[8 ] start
TIC TLS Event [8:0x1c036a080]: 1, Pending(0)
TIC TLS Event [8:0x1c036a080]: 2, Pending(0)
TIC TLS Event [8:0x1c036a080]: 11, Pending(0)
TIC TLS Event [8:0x1c036a080]: 12, Pending(0)
TIC TLS Event [8:0x1c036a080]: 14, Pending(0)
TIC TLS Trust Result [8:0x1c036a080]: 0
0x10bcb4000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x105805b00, main = 1)
Memory usage info dump at MainFrameLoadCompleted:
document_count: 56
virtual_size: 1918713856
internal: 81920000
javascript_gc_heap_capacity: 11894816
resident_size: 134414336
phys_footprint: 80200544
pagecache_page_count: 0
compressed: 0
javascript_gc_heap_extra_memory_size: 33952
TIC TLS Event [8:0x1c036a080]: 20, Pending(0)
TIC TCP Conn Connected [8:0x1c036a080]: Err(16)
TIC TCP Conn Event [8:0x1c036a080]: 1
TIC TCP Conn Event [8:0x1c036a080]: 8
TIC TLS Handshake Complete [8:0x1c036a080]
Task <640DC1A5-F732-4783-88F7-41FBCD5300B0>.<1> now using Connection 8
Task <640DC1A5-F732-4783-88F7-41FBCD5300B0>.<1> sent request, body N
Memory usage info dump at MainFrameLoadStarted:
document_count: 56
virtual_size: 1917190144
internal: 82034688
javascript_gc_heap_capacity: 11894816
resident_size: 134529024
phys_footprint: 80315232
pagecache_page_count: 0
compressed: 0
javascript_gc_heap_extra_memory_size: 33952
0x15de475c0 - FrameLoader::prepareForLoadStart: Starting frame load (frame = 0x105805b00, main = 1)
0x10c1b3000 - DocumentLoader::startLoadingMainResource: Starting load (frame = 0x105805b00, main = 1)
Task <640DC1A5-F732-4783-88F7-41FBCD5300B0>.<1> received response, status 200 content K
Task <640DC1A5-F732-4783-88F7-41FBCD5300B0>.<1> response ended
Memory usage info dump at MainFrameLoadCompleted:
document_count: 57
virtual_size: 1919926272
internal: 85131264
javascript_gc_heap_capacity: 19096466
resident_size: 137789440
phys_footprint: 83411808
pagecache_page_count: 0
compressed: 0
javascript_gc_heap_extra_memory_size: 5863362
0x15de475c0 - FrameLoader::checkLoadCompleteForThisFrame: Finished frame load (frame = 0x105805b00, main = 1)
0x1092bf000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10e4ff840, main = 0)
0x10bc3b000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x108bd5200, main = 0)
0x10bc3c000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10964ed00, main = 0)
0x1089b3000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10874f180, main = 0)
0x10bc32000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10e4ffcc0, main = 0)
Task <0CBA26F2-DC29-46BA-A3A6-E5BFD52547B9>.<0> now using Connection 1
Task <0CBA26F2-DC29-46BA-A3A6-E5BFD52547B9>.<0> sent request, body N
Task <0CBA26F2-DC29-46BA-A3A6-E5BFD52547B9>.<0> received response, status 200 content K
Task <0CBA26F2-DC29-46BA-A3A6-E5BFD52547B9>.<0> response ended
0x10a9de000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10a9c4d80, main = 0)
0x10a9dc000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10a9c5680, main = 0)
0x10a9be000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10a9c61c0, main = 0)
Task <53BFDEC1-2204-4EE6-811E-383F0B19885E>.<0> now using Connection 7
Task <53BFDEC1-2204-4EE6-811E-383F0B19885E>.<0> sent request, body N
0x10a9bc000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10a9c6ac0, main = 0)
Task <53BFDEC1-2204-4EE6-811E-383F0B19885E>.<0> received response, status 204 content K
Task <53BFDEC1-2204-4EE6-811E-383F0B19885E>.<0> response ended
0x10a98e000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10a9c7a80, main = 0)
TIC Enabling TLS [9:0x1c4364bc0]
TIC TCP Conn Start [9:0x1c4364bc0]
Task <68C96273-05D3-4F2D-A056-081027236ACD>.<0> setting up Connection 9
[9 ] start
0x10a98f000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10a9c73c0, main = 0)
0x1089f5000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x1089cc240, main = 0)
TIC TLS Event [9:0x1c4364bc0]: 1, Pending(0)
TIC TLS Event [9:0x1c4364bc0]: 2, Pending(0)
TIC TLS Event [9:0x1c4364bc0]: 11, Pending(0)
TIC TLS Event [9:0x1c4364bc0]: 12, Pending(0)
TIC TLS Event [9:0x1c4364bc0]: 14, Pending(0)
TIC TLS Trust Result [9:0x1c4364bc0]: 0
TIC TLS Event [9:0x1c4364bc0]: 20, Pending(0)
TIC TCP Conn Connected [9:0x1c4364bc0]: Err(16)
TIC TCP Conn Event [9:0x1c4364bc0]: 1
TIC TCP Conn Event [9:0x1c4364bc0]: 8
TIC TLS Handshake Complete [9:0x1c4364bc0]
Task <68C96273-05D3-4F2D-A056-081027236ACD>.<0> now using Connection 9
Task <68C96273-05D3-4F2D-A056-081027236ACD>.<0> sent request, body N
Task <68C96273-05D3-4F2D-A056-081027236ACD>.<0> received response, status 200 content K
Task <68C96273-05D3-4F2D-A056-081027236ACD>.<0> response ended
0x1089f4000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x1089cdd40, main = 0)
client.trigger:#N Random sample for 0x350002 is skip
client.trigger:#N Random sample for 0x350002 is skip
Task .<0> now using Connection 7
Task .<0> sent request, body N
Task .<0> received response, status 204 content K
Task .<0> response ended
Task <82D65FE3-4559-47FB-A0AE-900E60B6C88E>.<0> now using Connection 7
Task <82D65FE3-4559-47FB-A0AE-900E60B6C88E>.<0> sent request, body N
Task <82D65FE3-4559-47FB-A0AE-900E60B6C88E>.<0> received response, status 204 content K
Task <82D65FE3-4559-47FB-A0AE-900E60B6C88E>.<0> response ended
Task <91E267DF-B652-4F42-A6C4-861CBAE2BBD1>.<0> now using Connection 1
Task <91E267DF-B652-4F42-A6C4-861CBAE2BBD1>.<0> sent request, body N
Task <91E267DF-B652-4F42-A6C4-861CBAE2BBD1>.<0> received response, status 200 content K
Task <91E267DF-B652-4F42-A6C4-861CBAE2BBD1>.<0> response ended
client.trigger:#N Random sample for 0x350002 is skip
TIC Enabling TLS [10:0x1c036c480]
TIC TCP Conn Start [10:0x1c036c480]
Task .<2> setting up Connection 10
[10 ] start
TIC TLS Event [10:0x1c036c480]: 1, Pending(0)
TIC TLS Event [10:0x1c036c480]: 2, Pending(0)
TIC TLS Event [10:0x1c036c480]: 11, Pending(0)
TIC TLS Event [10:0x1c036c480]: 12, Pending(0)
TIC TLS Event [10:0x1c036c480]: 14, Pending(0)
TIC TLS Trust Result [10:0x1c036c480]: 0
TIC TLS Event [10:0x1c036c480]: 20, Pending(0)
TIC TCP Conn Connected [10:0x1c036c480]: Err(16)
TIC TCP Conn Event [10:0x1c036c480]: 1
TIC TCP Conn Event [10:0x1c036c480]: 8
TIC TLS Handshake Complete [10:0x1c036c480]
Task .<2> now using Connection 10
Task .<2> sent request, body N
Task .<2> received response, status 204 content U
Task .<2> response ended
Task <97531478-7F26-43A7-A40E-49529D03B4FF>.<0> now using Connection 7
Task <97531478-7F26-43A7-A40E-49529D03B4FF>.<0> sent request, body N
Task <97531478-7F26-43A7-A40E-49529D03B4FF>.<0> received response, status 204 content K
Task <97531478-7F26-43A7-A40E-49529D03B4FF>.<0> response ended
Task .<0> now using Connection 1
Task .<0> sent request, body N
Task .<0> received response, status 200 content K
Task .<0> response ended
client.trigger:#N Random sample for 0x350002 is skip
Task .<0> now using Connection 1
Task .<0> sent request, body N
Task .<0> received response, status 200 content K
Task .<0> response ended
client.trigger:#N Random sample for 0x350002 is skip
Task <1E2C6140-F11F-4069-A1A6-48F8F7550330>.<0> now using Connection 7
Task <1E2C6140-F11F-4069-A1A6-48F8F7550330>.<0> sent request, body N
CONSOLE LOG file:///app/main-view-model.js:36:28: onAdClosed called
Task <1E2C6140-F11F-4069-A1A6-48F8F7550330>.<0> received response, status 204 content K
Task <1E2C6140-F11F-4069-A1A6-48F8F7550330>.<0> response ended
0x1089f6000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x1089cd440, main = 0)
Task <66AF0C7C-F172-4FF5-B20D-1B99F68115C1>.<2> now using Connection 3
Task <66AF0C7C-F172-4FF5-B20D-1B99F68115C1>.<2> sent request, body N
Task <66AF0C7C-F172-4FF5-B20D-1B99F68115C1>.<2> received response, status 200 content K
Task <66AF0C7C-F172-4FF5-B20D-1B99F68115C1>.<2> response ended
0x10f4c9000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x1089cf3c0, main = 0)
0x10bcb6000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x105806400, main = 1)
Memory usage info dump at MainFrameLoadCompleted:
document_count: 72
virtual_size: 1939177472
internal: 87212032
javascript_gc_heap_capacity: 18380993
resident_size: 142983168
phys_footprint: 84165472
pagecache_page_count: 0
compressed: 0
javascript_gc_heap_extra_memory_size: 5901553
Memory usage info dump at MainFrameLoadStarted:
document_count: 72
virtual_size: 1939308544
internal: 87392256
javascript_gc_heap_capacity: 18380993
resident_size: 143163392
phys_footprint: 84345696
pagecache_page_count: 0
compressed: 0
javascript_gc_heap_extra_memory_size: 5901553
0x15ddf8bf0 - FrameLoader::prepareForLoadStart: Starting frame load (frame = 0x105806400, main = 1)
0x10f4cb000 - DocumentLoader::startLoadingMainResource: Returning cached main resource (frame = 0x105806400, main = 1)
0x110938000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x11093c000, main = 0)
Task <5C35569C-193B-4737-8F6F-0780A74668E9>.<0> now using Connection 4
Task <5C35569C-193B-4737-8F6F-0780A74668E9>.<0> sent request, body N
0x110cc5000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x11093e400, main = 0)
0x110cc7000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x11093ed00, main = 0)
0x110cc6000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x11093f600, main = 0)
0x110c89000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x11093c480, main = 0)
0x110c8b000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x11093e880, main = 0)
0x110c8a000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x110c80480, main = 0)
Task <5C35569C-193B-4737-8F6F-0780A74668E9>.<0> received response, status 200 content K
Task <5C35569C-193B-4737-8F6F-0780A74668E9>.<0> response ended
Memory usage info dump at MainFrameLoadCompleted:
document_count: 81
virtual_size: 1954283520
internal: 96616448
javascript_gc_heap_capacity: 22318701
resident_size: 152420352
phys_footprint: 93586312
pagecache_page_count: 0
compressed: 0
javascript_gc_heap_extra_memory_size: 5911565
0x15ddf8bf0 - FrameLoader::checkLoadCompleteForThisFrame: Finished frame load (frame = 0x105806400, main = 1)
0x110c88000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x110c81440, main = 0)
0x110c51000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x110c81d40, main = 0)
0x110c53000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x110c82640, main = 0)
0x110c52000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x110c82f40, main = 0)
0x110c50000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x110c83840, main = 0)
0x110939000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x110c821c0, main = 0)
0x110c39000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x110c83cc0, main = 0)
0x110c3b000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x110c2c240, main = 0)
0x110c3a000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x110c2cb40, main = 0)
0x110c38000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x110c2d440, main = 0)
0x110c1d000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x110c2dd40, main = 0)
0x110c1f000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x110c2e880, main = 0)
0x110c1e000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x110c2f180, main = 0)
0x110c1c000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x110c2fa80, main = 0)
Task <2E198B0B-C5C7-40EA-9E48-50D3E3B064DA>.<0> now using Connection 7
Task <2E198B0B-C5C7-40EA-9E48-50D3E3B064DA>.<0> sent request, body N
0x110ff9000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x110c80900, main = 0)
0x110ffb000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x110c2e1c0, main = 0)
0x10bc91000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10bc95200, main = 0)
TIC Enabling TLS [11:0x1c4366000]
TIC TCP Conn Start [11:0x1c4366000]
Task .<1> setting up Connection 11
[11 ] start
0x108bb1000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b823cc0, main = 0)
TIC TLS Event [11:0x1c4366000]: 1, Pending(0)
TIC TLS Event [11:0x1c4366000]: 2, Pending(0)
TIC TLS Event [11:0x1c4366000]: 11, Pending(0)
TIC TLS Event [11:0x1c4366000]: 12, Pending(0)
TIC TLS Event [11:0x1c4366000]: 14, Pending(0)
TIC TLS Trust Result [11:0x1c4366000]: 0
TIC TLS Event [11:0x1c4366000]: 20, Pending(0)
TIC TCP Conn Connected [11:0x1c4366000]: Err(16)
TIC TCP Conn Event [11:0x1c4366000]: 1
TIC TCP Conn Event [11:0x1c4366000]: 8
TIC TLS Handshake Complete [11:0x1c4366000]
Task .<1> now using Connection 11
Task .<1> sent request, body N
Task <2E198B0B-C5C7-40EA-9E48-50D3E3B064DA>.<0> received response, status 204 content K
Task <2E198B0B-C5C7-40EA-9E48-50D3E3B064DA>.<0> response ended
Task .<1> received response, status 303 content K
Task .<1> response ended
TIC Enabling TLS [12:0x1c0369240]
TIC TCP Conn Start [12:0x1c0369240]
Task .<1> setting up Connection 12
[12 ] start
TIC TLS Event [12:0x1c0369240]: 1, Pending(0)
TIC TLS Event [12:0x1c0369240]: 2, Pending(0)
TIC TLS Event [12:0x1c0369240]: 11, Pending(0)
TIC TLS Event [12:0x1c0369240]: 12, Pending(0)
TIC TLS Event [12:0x1c0369240]: 14, Pending(0)
TIC TLS Trust Result [12:0x1c0369240]: 0
TIC TLS Event [12:0x1c0369240]: 20, Pending(0)
TIC TCP Conn Connected [12:0x1c0369240]: Err(16)
TIC TCP Conn Event [12:0x1c0369240]: 1
TIC TCP Conn Event [12:0x1c0369240]: 8
TIC TLS Handshake Complete [12:0x1c0369240]
Task .<1> now using Connection 12
Task .<1> sent request, body N
Task .<1> received response, status 206 content K
Task <9E898430-0EE2-4EED-BC5C-7F7F20A73C39>.<0> now using Connection 7
Task <9E898430-0EE2-4EED-BC5C-7F7F20A73C39>.<0> sent request, body N
TIC TCP Conn Cancel [12:0x1c0369240]
Task .<1> response ended
[12 stream, pid: 2677, url: https://r1---sn-qxo7rn7e.googlevideo.com/videoplayback?id=d0ff911266dccf16&itag=22&source=youtube&requiressl=yes&mm=31&mn=sn-qxo7r, tls] cancelled
[12.1 CA24B142-39BD-40C5-891A-188B40B1EF39 .54659<->]
Connected Path: satisfied (Path is satisfied), interface: en0, ipv4, dns
Duration: 0.310s, DNS @0.003s took 0.009s, TCP @0.014s took 0.015s, TLS took 0.069s
bytes in/out: 1355374/1098, packets in/out: 983/3, rtt: 0.029s, retransmitted packets: 0, out-of-order packets: 0
TIC TCP Conn Destroyed [12:0x1c0369240]
TIC TCP Conn Cancel [11:0x1c4366000]
[11 stream, pid: 2677, url: https://www.youtube.com/get_video?video_id=0P-REmbczxY&ts=1552243221&t=g-2qskbqImy37uHL2cgRs5LakEk&gad=1&br=1&itag=22,18, tls] cancelled
[11.1 020FE922-C543-44AF-9BA6-351C7FBC75AE .54658<->]
Connected Path: satisfied (Path is satisfied), interface: en0, ipv4, dns
Duration: 0.489s, DNS @0.000s took 0.003s, TCP @0.004s took 0.015s, TLS took 0.048s
bytes in/out: 4629/885, packets in/out: 9/10, rtt: 0.021s, retransmitted packets: 1, out-of-order packets: 0
TIC TCP Conn Destroyed [11:0x1c4366000]
TIC Read Status [11:0x0]: 1:57
TIC Read Status [11:0x0]: 1:57
Task <9E898430-0EE2-4EED-BC5C-7F7F20A73C39>.<0> received response, status 204 content K
Task <9E898430-0EE2-4EED-BC5C-7F7F20A73C39>.<0> response ended
client.trigger:#N Random sample for 0x350002 is skip
client.trigger:#N Random sample for 0x350002 is skip
Task <4A2C7B00-77A2-4C45-A222-7CEC7CDF949E>.<0> now using Connection 7
Task <4A2C7B00-77A2-4C45-A222-7CEC7CDF949E>.<0> sent request, body N
Task <4A2C7B00-77A2-4C45-A222-7CEC7CDF949E>.<0> received response, status 204 content K
Task <4A2C7B00-77A2-4C45-A222-7CEC7CDF949E>.<0> response ended
Task .<3> now using Connection 8
Task .<3> sent request, body N
Task .<3> received response, status 200 content K
Task .<3> response ended
0x10bc90000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b8206c0, main = 0)
0x10b8e8000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b820fc0, main = 0)
0x110ff8000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b8218c0, main = 0)
0x10b8e9000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b8221c0, main = 0)
0x1089b0000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b8cd200, main = 0)
0x110f89000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b8cdb00, main = 0)
Task .<0> now using Connection 1
Task .<0> sent request, body N
Task .<0> received response, status 200 content K
Task .<0> response ended
0x110f8b000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b8ce1c0, main = 0)
0x110f88000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10bc94240, main = 0)
0x110f4e000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b820b40, main = 0)
Task .<0> now using Connection 9
Task .<0> sent request, body N
0x110f4c000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b8cfa80, main = 0)
Task <0FEADFC9-9430-4E3D-997D-6F1FB3F71CDA>.<0> now using Connection 7
Task <0FEADFC9-9430-4E3D-997D-6F1FB3F71CDA>.<0> sent request, body N
0x110f4f000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b821440, main = 0)
Task .<0> received response, status 200 content K
Task <0FEADFC9-9430-4E3D-997D-6F1FB3F71CDA>.<0> received response, status 204 content K
Task <0FEADFC9-9430-4E3D-997D-6F1FB3F71CDA>.<0> response ended
Task .<0> response ended
0x110f32000 - DocumentLoader::startLoadingMainResource: Returning empty document (frame = 0x10b823a80, main = 0)
TIC TCP Conn Cancel [2:0x1c417de80]
[2 stream, pid: 2677, url: https://googleads.g.doubleclick.net/mads/static/mad/sdk/native/sdk-core-v40-loader.appcache, tls] cancelled
[2.1 11755975-D324-4423-9B0C-2416A9DEE845 .54649<->]
Connected Path: satisfied (Path is satisfied), interface: en0, ipv4, dns
Duration: 30.962s, DNS @0.001s took 0.004s, TCP @0.011s took 0.016s, TLS took 0.030s
bytes in/out: 1042/820, packets in/out: 2/3, rtt: 0.030s, retransmitted packets: 0, out-of-order packets: 0
Task <817141F9-64BD-4C3E-AE01-8A124AA9A78B>.<0> now using Connection 7
Task <817141F9-64BD-4C3E-AE01-8A124AA9A78B>.<0> sent request, body N
client.trigger:#N Random sample for 0x350002 is skip
client.trigger:#N Random sample for 0x350002 is skip
Task <817141F9-64BD-4C3E-AE01-8A124AA9A78B>.<0> received response, status 204 content K
Task <817141F9-64BD-4C3E-AE01-8A124AA9A78B>.<0> response ended
Task .<0> now using Connection 7
Task .<0> sent request, body N
Task .<0> received response, status 204 content K
Task .<0> response ended
Task .<0> now using Connection 1
Task .<0> sent request, body N
Task .<0> received response, status 200 content K
Task .<0> response ended
client.trigger:#N Random sample for 0x350002 is skip
Task <60B46E8F-672B-4B73-ABF2-F2E02C91BEB1>.<4> now using Connection 10
Task <60B46E8F-672B-4B73-ABF2-F2E02C91BEB1>.<4> sent request, body N
Task <60B46E8F-672B-4B73-ABF2-F2E02C91BEB1>.<4> received response, status 204 content U
Task <60B46E8F-672B-4B73-ABF2-F2E02C91BEB1>.<4> response ended
Task .<0> now using Connection 7
Task .<0> sent request, body N
Task .<0> received response, status 204 content K
Task .<0> response ended
Task <2295D9CC-3DAD-4C3A-BF5E-473A869B10B0>.<0> now using Connection 1
Task <2295D9CC-3DAD-4C3A-BF5E-473A869B10B0>.<0> sent request, body N
Task <2295D9CC-3DAD-4C3A-BF5E-473A869B10B0>.<0> received response, status 200 content K
Task <2295D9CC-3DAD-4C3A-BF5E-473A869B10B0>.<0> response ended
Task <8A065411-C833-4BC5-AB84-63F496DED3B5>.<0> now using Connection 7
Task <8A065411-C833-4BC5-AB84-63F496DED3B5>.<0> sent request, body N
Task <8A065411-C833-4BC5-AB84-63F496DED3B5>.<0> received response, status 204 content K
Task <8A065411-C833-4BC5-AB84-63F496DED3B5>.<0> response ended
client.trigger:#N Random sample for 0x350002 is skip
TIC TCP Conn Cancel [4:0x1c0367800]
[4 stream, pid: 2677, url: https://lh3.googleusercontent.com/1vSwOAGw8Btx-k16b48dN15KRZnhBV7gmw-YRO7k46V7l7g6tQnaIkm6G9uBj7FldthqH-i2Pg=s124-l80#fifeurl, tls] cancelled
[4.1 E9E13119-1EFE-4F40-BC06-012604DF2379 .54651<->]
Connected Path: satisfied (Path is satisfied), interface: en0, ipv4, dns
Duration: 53.588s, DNS @0.000s took 0.005s, TCP @0.007s took 0.017s, TLS took 0.074s
bytes in/out: 17739/2099, packets in/out: 15/4, rtt: 0.018s, retransmitted packets: 0, out-of-order packets: 0
TIC TCP Conn Cancel [9:0x1c4364bc0]
[9 stream, pid: 2677, url: https://pagead2.googlesyndication.com/pcs/activeview?xai=AKAOjssIR0RAToMDmMPBBo-l5PhQ-1lBjvFIN45NzPYxuhzsK2-nFG1MDalRNDpjZvcGJqly0, tls] cancelled
[9.1 84CC810D-767A-4006-A944-B50E5407017D .54656<->]
Connected Path: satisfied (Path is satisfied), interface: en0, ipv4, dns
Duration: 56.152s, DNS @0.001s took 0.008s, TCP @0.013s took 0.015s, TLS took 0.102s
bytes in/out: 4152/3085, packets in/out: 5/6, rtt: 0.016s, retransmitted packets: 0, out-of-order packets: 0
TIC TCP Conn Cancel [1:0x1c017cf80]
[1 stream, pid: 2677, url: https://googleads.g.doubleclick.net/mads/static/sdk/native/sdk-core-v40.html?sdk=afma-sdk-i-v7.37.0, tls] cancelled
[1.1 D84F346D-2407-4F45-96A0-CC970CD85E38 .54648<->]
Connected Path: satisfied (Path is satisfied), interface: en0, ipv4, dns
Duration: 71.967s, DNS @0.000s took 0.017s, TCP @0.034s took 0.016s, TLS took 0.085s
bytes in/out: 123275/14998, packets in/out: 96/21, rtt: 0.018s, retransmitted packets: 0, out-of-order packets: 0
TIC TCP Conn Cancel [7:0x1c4364080]
[7 stream, pid: 2677, url: https://csi.gstatic.com/csi?v=3&s=gmob&action=js_webview_partial&e=318477469,318483349,318484345,318483174,318483670,318484156,318, tls] cancelled
[7.1 37A0A972-963A-4573-A3E7-58398F930CAF .54654<->]
Connected Path: satisfied (Path is satisfied), interface: en0, ipv4, dns
Duration: 70.706s, DNS @0.001s took 0.020s, TCP @0.022s took 0.144s, TLS took 0.320s
bytes in/out: 9620/27323, packets in/out: 19/31, rtt: 0.253s, retransmitted packets: 0, out-of-order packets: 0
Final notes
I will submit a PR if I find a solution, in the meantime, any extra eyes are appreciated.