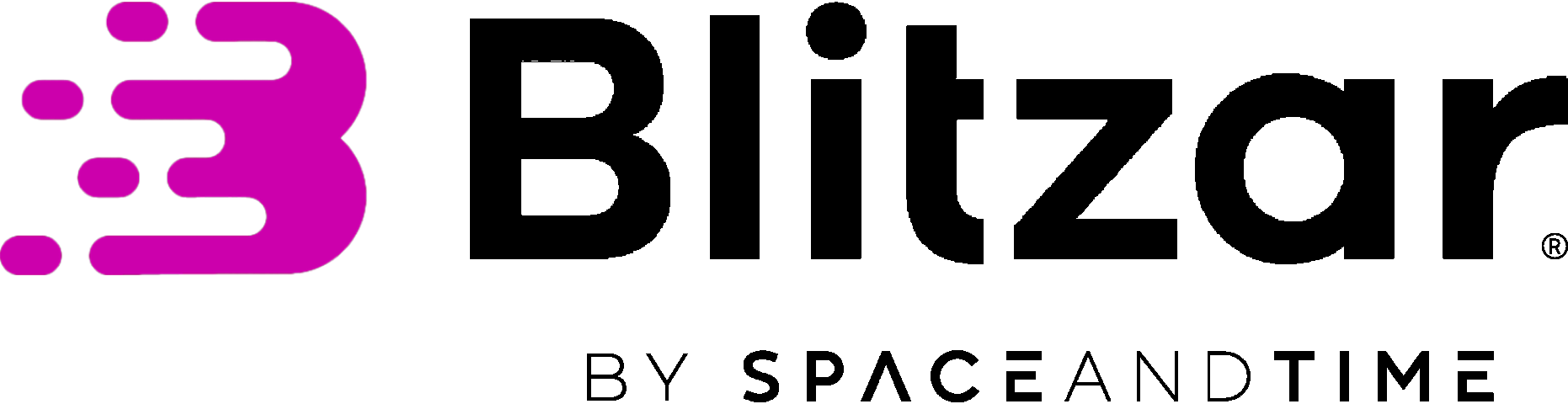
Space and Time C++ library for accelerating cryptographic zero-knowledge proofs algorithms on the CPU and GPU.
Report Bug
|
Request a Feature
Blitzar was created by the core cryptography team at Space and Time to accelerate Proof of SQL, a novel zero-knowledge proof for SQL operations. After surveying our options for a GPU acceleration framework, we realized that Proof of SQL needed something better… so we built Blitzar. Now, Proof of SQL runs with a 3.2 second proving time against a million-row table on a single GPU, and it’s only getting faster.
We’ve open-sourced Blitzar to provide the Web3 community with a faster and more robust framework for building GPU-accelerated zk-proofs. We’re excited to open the project to community contributions to expand the scope of Blitzar and lay the foundation for the next wave of lightning fast zk-proofs.
Blitzar is a C++ library for accelerating cryptographic zero-knowledge proof algorithms on the CPU and GPU.
Note
This repo contains the C++ implementation along with cbindings and a Rust sys-crate. If you are using Rust, use the crate from the companion blitzar-rs repo.
The library provides
- Functions for doing group operations on Curve-25519 and Ristretto25519 elements.
- An implementation of Inner Product Argument Protocol for producing and verifying a compact proof of the inner product of two vectors.
- A sys-crate and bindings to make commitment computations usable from Rust.
The library is adopted from code in libsodium and extends libsodium's cryptographic functions to support CUDA so that they are usable on GPUs.
WARNING: This project has not undergone a security audit and is NOT ready for production use.
Although the primary goal of this library is to provide GPU acceleration for cryptographic zk-proof algorithms, the library also provides CPU support for the sake of testing. The following backends are supported:
Backend | Implementation | Target Hardware |
---|---|---|
cpu |
Serial | x86 capable CPUs |
gpu |
Parallel | Nvidia CUDA capable GPUs |
Blitzar provides an implementation of Multi-Scalar Multiplication (i.e. generalized Pedersen commitments)
Let
Then, the Generalized Pedersen Commitment of the vector
Note: we interchangeably use the terms "multi-scalar multiplication" and "multiexponentiation" to refer to the this operation because when the group is written additively, the operation is a multi-scalar multiplication, and when the group is written multiplicatively, the operation is a multiexponentiation.
The Blitzar implementation allows for computation of multiple, potentially different length, MSMs simultaneously. Additionally, either built-in, precomputed, generators
Currently, Blitzar supports Curve25519 as the group. We're always working to expand the curves that we support, so check back for updates.
Blitzar provides a modified implementation of an inner product argument (e.g. Bulletproofs and Halo2).
Given generators
where it is assumed that
This version of the inner product argument can be used in the context of a broader protocol.
If there is a particular feature that you would like to see, please reach out. Blitzar is a community-first project, and we want to hear from you.
Performance (associated commit hash)
Benchmarks are run against four different types of GPU:
- Nvidia 3060
- Nvidia T4 - Standard_NC4as_T4_v3
- Nvidia V100 - Standard_NC6s_v3
- Nvidia A100 - Standard_NC24ads_A100_v4
Multi-Scalar Multiplication / Generalized Pedersen Commitment Results:
The subsequent outcomes are derived from the preceding benchmark execution of the pedersen commitment, during which the number of sequences, bytes per element, sequence length, and GPU type were varied.
Inner Product Argument Results:
The subsequent outcomes are derived from the preceding benchmark execution of the inner product, during which the number of elements and the type of GPU were changed.
See the example folder for some examples.
Build environment
Prerequisites:
x86_64
Linux instance.- Nix with flake support (check out The Determinate Nix Installer)
- Nvidia GPU capable of running CUDA 12.2 code.
From your terminal, run the following command in the root of the source directory to set up a build environment.
nix develop
Note: if this is the first time, it may take a while as we build a clang compiler from source.
Building and Testing the C++/CUDA code:
nix develop
# build all the code assets
bazel build //...
# run all tests
bazel test //...
# run all memory sanitized tests
bazel test --config=asan //...
Note: some tests will fail in case you don't have a GPU available.
Building and Testing the Rust Sys-Crate code:
nix develop
# run the sys-crate tests
cargo test --manifest-path rust/blitzar-sys/Cargo.toml
Although possible, this sys-crate is not meant to be used directly by Rust users. Instead, consider using the blitzar-rs, which is a high-level wrapper around this sys-crate.
Note: the shared library byproduct of the C++/CUDA code is automatically copied to the Rust sys-crate under the rust/blitzar-sys/
directory.
You can find release ready versions of this library under the release page. You can also build and test the library from source by following the instructions below.
C++ Project:
See the C++ example here: example/cbindings1/main.cc. To run this example, execute:
nix develop
bazel run //example/cbindings1:cbind1
Alternatively, compile this example code with g++:
nix develop
# Build the shared library
bazel build -c opt --config=portable_glibc //cbindings:libblitzar.so
# Compile the C++ example code
g++ example/cbindings1/main.cc -o main -I . -L bazel-bin/cbindings/ -lblitzar
# Execute the example code
LD_LIBRARY_PATH=$LD_LIBRARY_PATH:bazel-bin/cbindings/ ./main
Rust Project:
See the Rust example here: rust/tests/src/main.rs. To run this example, execute:
nix develop
cargo test --manifest-path rust/tests/Cargo.toml
The main branch is regularly built and tested, being the only source of truth. Tags are created regularly from automated semantic release executions.
This repository follows some C++ formatting rules. To check if your modified code is compliant, run the following commands:
nix develop
./tools/code_format/check_format.py check
./tools/code_format/check_format.py fix
Generalized Pedersen Commitment (MSM):
nix develop
# Usage: benchmark <cpu|gpu> <n> <num_samples> <num_commitments> <element_nbytes> <verbose>
# - n: the size of the multiexponentiation vector (or the sequence length).
# - num_samples: the number of times to run the benchmark.
# - num_commitments: the number of commitments to generate.
# - element_nbytes: the number of bytes of each element in the vector (exponent size).
# - verbose: whether to print the results of each run.
bazel run -c opt //benchmark/multi_commitment:benchmark -- gpu 1000 5 1 32 1
Inner Product Argument:
nix develop
# Usage: benchmark <cpu|gpu> <n> <num_samples>
# - n: the size of the inner product vector (or the sequence length).
# - num_samples: the number of times to run the benchmark.
bazel run -c opt //benchmark/inner_product_proof:benchmark -- gpu 1000 5
We're excited to open Blitzar to the community, but are not accepting community Pull Requests yet due to logistic reasons. However, feel free to contribute with any suggestion, idea, or bugfix on our Issues panel. Also, see contribution guide.
Join our Discord server to ask questions, discuss features, and for general chat.
This project is released under the Apache 2 License.
This repo contains the C++ implementation along with cbindings and a Rust sys-crate. If you are using Rust, use the crate from the companion repo here: https://github.com/spaceandtimelabs/blitzar-rs.