Describe the bug
A clear and concise description of what the bug is.
Working off the flocking example seek to change color of agents when they are close and reset when the distance. I cannot get to change the color of the agents, and I am getting an error on the console saying the color is None.
Please advise, what I am doing wrong. Attaching code and screen shot. The agents are very close but color does not change. (Ubuntu 14.04, Mozilla Firefox 66.0.3)
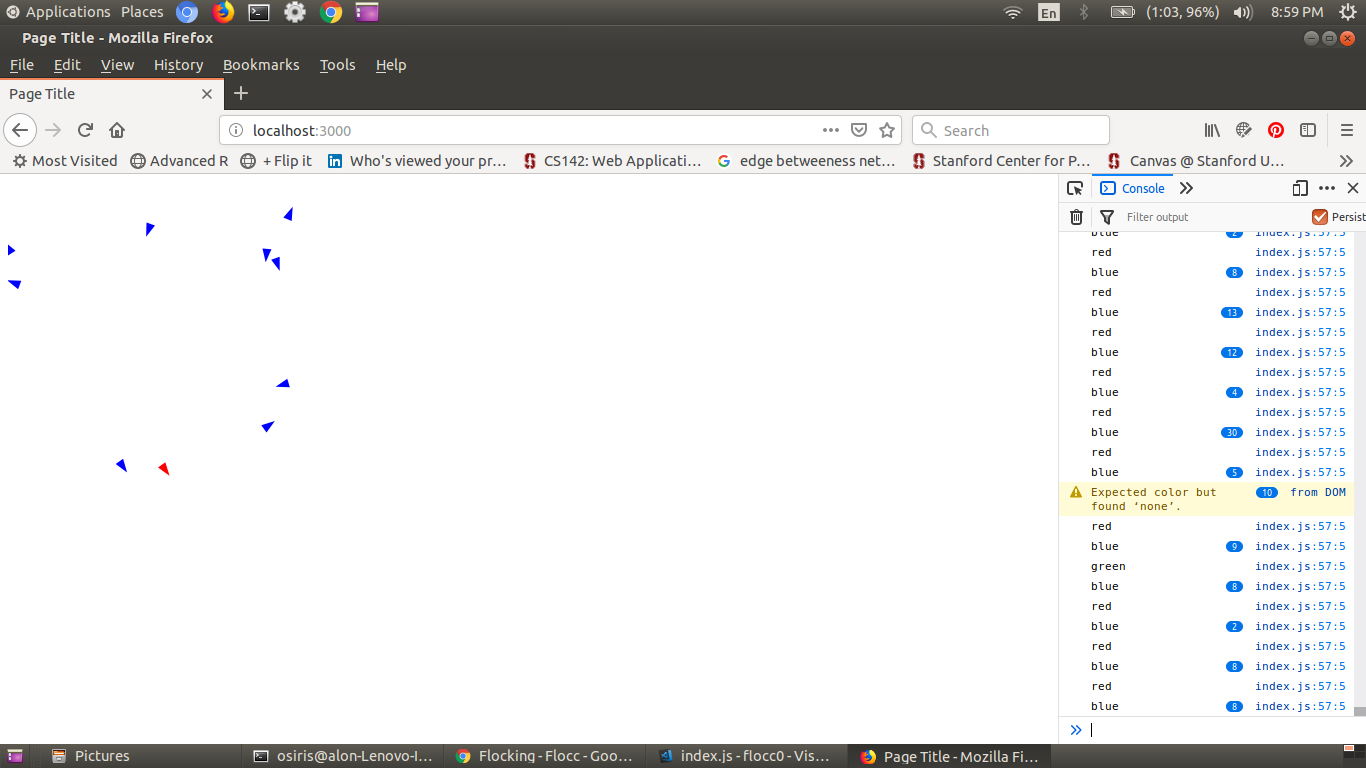
`const { Agent, Environment, GridEnvironment, ASCIIRenderer, CanvasRenderer, utils, Vector } = flocc;
/* ------- PARAMETERS --------- */
const flockSize = 10;
const width = 300;
const height = 300;
/* ---------------------------- */
/* ------- SET UP ENVIRONMENT, RENDERER --------- */
const environment = new Environment();
const renderer = new CanvasRenderer(environment, { width, height });
const container = document.getElementById('container');
console.log(container)
renderer.mount(container);
function setup() {
for (let i = 0; i < flockSize; i++) {
const agent = new Agent();
agent.set('x', 0.25 * Math.random() * width);
agent.set('y', 0.25 * Math.random() * height);
agent.set('shape', 'arrow');
agent.set('color', '#0F0');
agent.set('size', 3);
const angle = 2 * Math.random() * Math.PI;
agent.set('vx', Math.cos(angle));
agent.set('vy', Math.sin(angle));
agent.addRule(tick);
environment.addAgent(agent);
}
}
function tick(agent) {
var x = agent.get('x');
var y = agent.get('y');
x += agent.get('vx');
y += agent.get('vy');
while (x < 0) x += width;
while (x >= width) x -= width;
while (y < 0) y += height;
while (y >= height) y -= height;
agent.set('x', x);
agent.set('y', y);
// update color if the agents are close
environment.getAgents().forEach(function(x){
console.log(x.get('color'))
})
environment.getAgents().filter(function(other){
var d = utils.distance(agent,other);
if ( d<=60 & d >0 ){
other.set('color','red');
agent.set('color','green');
} else {
other.set('color','blue');
agent.set('color','blue');
}
});
}
function render() {
environment.tick();
requestAnimationFrame(render);
}
setup();
render();
`
To Reproduce
Steps to reproduce the behavior:
- Go to '...'
- Click on '....'
- Scroll down to '....'
- See error
Expected behavior
A clear and concise description of what you expected to happen.
Screenshots
If applicable, add screenshots to help explain your problem.
Desktop (please complete the following information):
- OS: [e.g. iOS]
- Browser [e.g. chrome, safari]
- Version [e.g. 22]
Smartphone (please complete the following information):
- Device: [e.g. iPhone6]
- OS: [e.g. iOS8.1]
- Browser [e.g. stock browser, safari]
- Version [e.g. 22]
Additional context
Add any other context about the problem here.